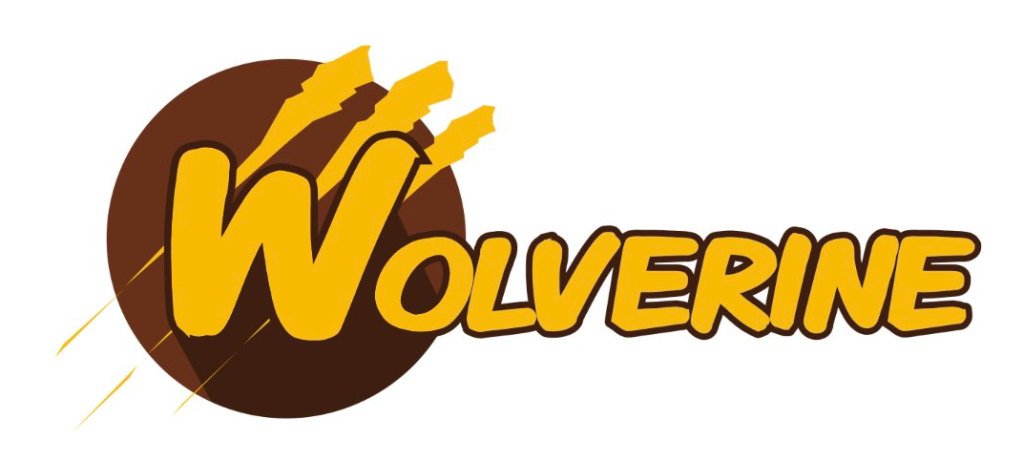
TL;DR: Wolverine can enable you to write testable code and achieve separation of concerns in your server side code with far less code ceremony than typical Clean Architecture type approaches.
I’m part of the mini-backlash against heavyweight, prescriptively layered architectural patterns like the various flavors of Hexagonal Architecture. I even did a whole talk on that subject at NDC Oslo this year:
Instead, I’m a big fan of keeping closely related code closer together with something like what Jimmy Bogard coined as Vertical Slices. Conveniently enough, I happen to think that Wolverine is a good fit for that style.
From a conference talk I did early last year, I started to build out a sample “TeleHealth Portal” system using the full “critter stack” with both Marten for persistence and event sourcing and Wolverine for everything else. Inside of this fictional TeleHealth system there will be a web service that adds a healthcare provider to an active board of related appointment requests (as an example, you might have a board for pediatric appointments in the state of Texas). When this web service executes, it needs to:
- Find the related information about the requested, active
Board
and theProvider
- Validate that the provider in question is able to join the active board based on various business rules like “is this provider licensed in this particular state and for some specialty?”. If the validation fails, the web service should return the validation message with the ProblemDetails specification
- Assuming the validation is good, start a new event stream with Marten for a
ProviderShift
that will track what the provider does during their active shift on that board for that specific day
I’ll need to add a little more context afterward for some application configuration, but here’s that functionality in one single Wolverine.Http endpoint class — with the assumption that the heavy duty business logic for validating the provider & board assignment is in the business domain model:
public record StartProviderShift(Guid BoardId, Guid ProviderId);
public record ShiftStartingResponse(Guid ShiftId) : CreationResponse("/shift/" + ShiftId);
public static class StartProviderShiftEndpoint
{
// This would be called before the method below
public static async Task<(Board, Provider, IResult)> LoadAsync(StartProviderShift command, IQuerySession session)
{
// You could get clever here and batch the queries to Marten
// here, but let that be a later optimization step
var board = await session.LoadAsync<Board>(command.BoardId);
var provider = await session.LoadAsync<Provider>(command.ProviderId);
if (board == null || provider == null) return (board, provider, Results.BadRequest());
// This just means "full speed ahead"
return (board, provider, WolverineContinue.Result());
}
[WolverineBefore]
public static IResult Validate(Provider provider, Board board)
{
// Check if you can proceed to add the provider to the board
// This logic is out of the scope of this sample:)
if (provider.CanJoin(board))
{
// Again, this value tells Wolverine to keep processing
// the HTTP request
return WolverineContinue.Result();
}
// No soup for you!
var problems = new ProblemDetails
{
Detail = "Provider is ineligible to join this Board",
Status = 400,
Extensions =
{
[nameof(StartProviderShift.ProviderId)] = provider.Id,
[nameof(StartProviderShift.BoardId)] = board.Id
}
};
// Wolverine will execute this IResult
// and stop all other HTTP processing
return Results.Problem(problems);
}
[WolverinePost("/shift/start")]
// In the tuple that's returned below,
// The first value of ShiftStartingResponse is assumed by Wolverine to be the
// HTTP response body
// The subsequent IStartStream value is executed as a side effect by Wolverine
public static (ShiftStartingResponse, IStartStream) Create(StartProviderShift command, Board board, Provider provider)
{
var started = new ProviderJoined(board.Id, provider.Id);
var op = MartenOps.StartStream<ProviderShift>(started);
return (new ShiftStartingResponse(op.StreamId), op);
}
}
And there’s a few things I’d ask you to notice in the code above:
- It’s just one class in one file that’s largely using functional decomposition to establish separation of concerns
- Wolverine.Http is able to call the various methods in order from top to bottom, pass the loaded data from
LoadAsync()
toValidate()
and on finally to theCreate()
method - I didn’t bother with any kind of repository abstraction around the data loading in the first step
- The
Validate()
method is a pure function that’s suitable for easy unit testing of the validation logic - The
Create()
method is also a pure, synchronous function that’s going to be easy to unit test as you can do assertions on the events contained in theIStartStream
object - Wolverine’s Marten integration is able to do the actual persistence of the new event stream for
ProviderShift
for you and deal with all the icky asynchronous junk
For more context, here’s the (butt ugly) code that Wolverine generates for the HTTP endpoint:
public class POST_shift_start : Wolverine.Http.HttpHandler
{
private readonly Wolverine.Http.WolverineHttpOptions _options;
private readonly Marten.ISessionFactory _sessionFactory;
public POST_shift_start(Wolverine.Http.WolverineHttpOptions options, Marten.ISessionFactory sessionFactory) : base(options)
{
_options = options;
_sessionFactory = sessionFactory;
}
public override async System.Threading.Tasks.Task Handle(Microsoft.AspNetCore.Http.HttpContext httpContext)
{
await using var documentSession = _sessionFactory.OpenSession();
await using var querySession = _sessionFactory.QuerySession();
var (command, jsonContinue) = await ReadJsonAsync<TeleHealth.WebApi.StartProviderShift>(httpContext);
if (jsonContinue == Wolverine.HandlerContinuation.Stop) return;
(var board, var provider, var result) = await TeleHealth.WebApi.StartProviderShiftEndpoint.LoadAsync(command, querySession).ConfigureAwait(false);
if (!(result is Wolverine.Http.WolverineContinue))
{
await result.ExecuteAsync(httpContext).ConfigureAwait(false);
return;
}
var result = TeleHealth.WebApi.StartProviderShiftEndpoint.Validate(provider, board);
if (!(result is Wolverine.Http.WolverineContinue))
{
await result.ExecuteAsync(httpContext).ConfigureAwait(false);
return;
}
(var shiftStartingResponse, var startStream) = TeleHealth.WebApi.StartProviderShiftEndpoint.Create(command, board, provider);
// Placed by Wolverine's ISideEffect policy
startStream.Execute(documentSession);
((Wolverine.Http.IHttpAware)shiftStartingResponse).Apply(httpContext);
// Commit any outstanding Marten changes
await documentSession.SaveChangesAsync(httpContext.RequestAborted).ConfigureAwait(false);
await WriteJsonAsync(httpContext, shiftStartingResponse);
}
}
In the application bootstrapping, I have Wolverine applying transactional middleware automatically:
builder.Host.UseWolverine(opts =>
{
// more config...
// Automatic usage of transactional middleware as
// Wolverine recognizes that an HTTP endpoint or message handler
// persists data
opts.Policies.AutoApplyTransactions();
});
And the Wolverine/Marten integration configured as well:
builder.Services.AddMarten(opts =>
{
var connString = builder
.Configuration
.GetConnectionString("marten");
opts.Connection(connString);
// There will be more here later...
})
// I added this to enroll Marten in the Wolverine outbox
.IntegrateWithWolverine()
// I also added this to opt into events being forward to
// the Wolverine outbox during SaveChangesAsync()
.EventForwardingToWolverine();
I’ll even go farther and say that in many cases Wolverine will allow you to establish decent separation of concerns and testability with far less ceremony than is required today with high overhead approaches like the popular Clean Architecture style.
Nice article! Do you have the sample code you mentioned here in anu GitHub repository?
I banged that out fast the other day. Not yet, but I’ll start a new repo for it next week when I get around to the 2nd entry
That’d be awesome. Thanks a ton for your OSS work!