Hey, did you know that JasperFx Software is ready for formal support plans for Marten and Wolverine? Not only are we trying to make the “Critter Stack” tools be viable long term options for your shop, we’re also interested in hearing your opinions about the tools and how they should change. We’re also certainly open to help you succeed with your software development projects on a consulting basis whether you’re using any part of the Critter Stack or any other .NET server side tooling.
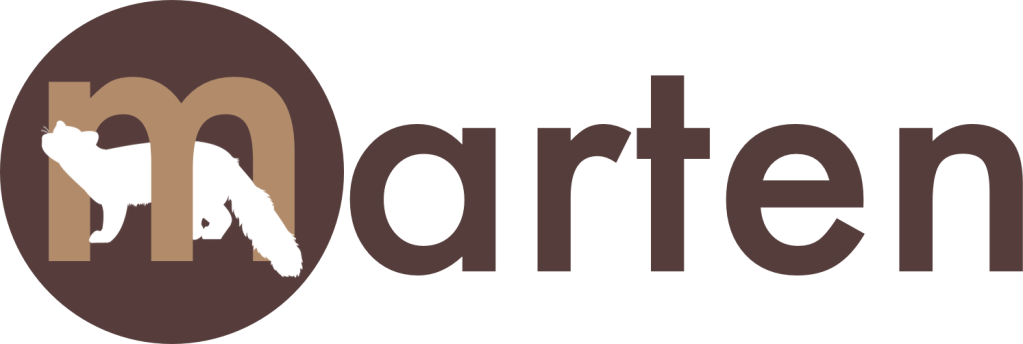
Let’s build a small web service application using the whole “Critter Stack” and their friends, one small step at a time. For right now, the “finished” code is at CritterStackHelpDesk on GitHub.
The posts in this series are:
- Event Storming
- Marten as Event Store
- Marten Projections
- Integrating Marten into Our Application
- Wolverine as Mediator
- Web Service Query Endpoints with Marten
- Dealing with Concurrency
- Wolverine’s Aggregate Handler Workflow FTW!
- Command Line Diagnostics with Oakton
- Integration Testing Harness
- Marten as Document Database (this post)
- Asynchronous Processing with Wolverine
- Durable Outbox Messaging and Why You Care!
- Wolverine HTTP Endpoints
- Easy Unit Testing with Pure Functions
- Vertical Slice Architecture
- Messaging with Rabbit MQ
- The “Stateful Resource” Model
- Resiliency
So far, we’ve been completely focused on using Marten as an Event Store. While the Marten team is very committed to the event sourcing feature set, it’s pretty likely that you’ll have other data persistence needs in your system that won’t fit the event sourcing paradigm. Not to worry though, because Marten also has a very robust “PostgreSQL as Document Database” feature set that’s perfect for low friction data persistence outside of the event storage. We’ve even used it in earlier posts as Marten projections utilize Marten’s document database features when projections are running Inline
or Async
(i.e., not Live
).
Since we’ve already got Marten integrated into our help desk application at this point, let’s just start with a document to represent customers:
public class Customer
{
public Guid Id { get; set; }
// We'll use this later for some "logic" about how incidents
// can be automatically prioritized
public Dictionary<IncidentCategory, IncidentPriority> Priorities { get; set; }
= new();
public string? Region { get; set; }
public ContractDuration Duration { get; set; }
}
public record ContractDuration(DateOnly Start, DateOnly End);
To be honest, I’m guessing at what a Customer
might involve in the end, but it’s okay that I don’t know that upfront per se because as we’ll see soon, Marten makes it very easy to evolve your persisted documents.
Having built the integration test harness for our application in the last post, let’s drop right into an integration test that persists a new Customer
document object, and reloads a copy from the persisted data:
public class using_customer_document : IntegrationContext
{
public using_customer_document(AppFixture fixture) : base(fixture)
{
}
[Fact]
public async Task persist_and_load_customer_data()
{
var customer = new Customer
{
Duration = new ContractDuration(new DateOnly(2023, 12, 1), new DateOnly(2024, 12, 1)),
Region = "West Coast",
Priorities = new Dictionary<IncidentCategory, IncidentPriority>
{
{ IncidentCategory.Database, IncidentPriority.High }
}
};
// As a convenience just because you'll use it so often in tests,
// I made a property named "Store" on the base class for quick access to
// the DocumentStore for the application
// ALWAYS remember to dispose any sessions you open in tests!
await using var session = Store.LightweightSession();
// Tell Marten to save the new document
session.Store(customer);
// commit any pending changes
await session.SaveChangesAsync();
// Marten is assigning an Id for you when one doesn't already
// exist, so that's where that value comes from
var copy = await session.LoadAsync<Customer>(customer.Id);
// Just proving to you that it's not the same object
copy.ShouldNotBeSameAs(customer);
copy.Duration.ShouldBe(customer.Duration);
}
}
As long as the configured database for our help desk API is available, the test above will happily pass. I’d like to draw your attention to a couple things about that test above:
- Notice that I didn’t have to make any changes to our application’s
AddMarten()
configuration in theProgram
file first because Marten is able to create storage for the newCustomer
document type on the fly when it first encounters it with its default settings - Marten is able to infer that the
Id
property of the newCustomer
type is the identity (that can be overridden), and when you add a newCustomer
document to the session that has an emptyGuid
as itsId
, Marten will quickly assign and set a sequential Guid value for its identity. If you’re wondering, Marten can do this even if the property is scoped asprivate
. - The
Store()
method is effectively an “upsert,” that takes advantage of PostgreSQL’s very efficient, built in upsert syntax. Marten does also supportInsert
andUpdate
operations, butStore
is just an easy default
Behind the scenes, Marten is just serializing our document to JSON and storing that data in a PostgreSQL JSONB column type that will allow for efficient querying within the JSON body later (if you’re immediately asking “why isn’t this thing supporting Sql Server?!?, it’s because only PostgreSQL has the JSONB type). If your document type can be round-tripped by either the venerable Newtonsoft.Json library or the newer System.Text.Json library, that document type can be persisted by Marten with zero explicit mapping.
In many cases, Marten’s approach to object persistence can lead to far less friction and boilerplate code than the equivalent functionality using EF Core, the .NET developer tool of choice. Moreover, using Marten requires a lot fewer database migrations as you change and evolve your document structure, giving developers far more ability to iterate over the shape of their persisted types as opposed to an ORM + Relational Database combination.
And of course, this is .NET, so Marten does come with LINQ support, so we can do queries like this:
var results = await session.Query<Customer>()
.Where(x => x.Region == "West Coast")
.OrderByDescending(x => x.Duration.End)
.ToListAsync();
As you’ll already know if you happen to follow me on Mastodon, we’re hopefully nearing the end of some very substantial improvements to the LINQ support for the forthcoming Marten v7 release.
While the document database feature set in Marten is pretty deep, the last thing I want to show in this post is that yes, you can create indexes within the JSON body for faster querying as needed. This time, I am going to the AddMarten()
configuration in the Program
file and add a little bit of code to index the Customer
document on its Region
field:
builder.Services.AddMarten(opts =>
{
// other configuration...
// This will create a btree index within the JSONB data
opts.Schema.For<Customer>().Index(x => x.Region);
});
Summary and What’s Next
Once upon a time, Marten started with a pressing need to have a reliable, ACID-compliant document database feature set, and we originally chose PostgreSQL because of its unique JSON feature set. Almost on a lark, I added a nascent event sourcing capability before the original Marten 1.0 release. To my surprise, the event sourcing feature set is the main driver of Marten adoption by far, but Marten still has its original feature set to make the rock solid PostgreSQL database engine function as a document database for .NET developers.
Even in a system using event sourcing, there’s almost always some kind of relatively static reference data that’s better suited for Marten’s document database feature set or even going back to using PostgreSQL as the outstanding relational database engine that it is.
In the next post, now that we also know how to store and retrieve customer documents with Marten, we’re going to introduce Wolverine’s “compound handler” capability and see how that can help us factor our code into being very testable.